With GPS receivers integrated in phones, tablets and PCs, a lot of apps are being built that displays your current location. Usually the GPS API’s that these devices come with provides you with two values: Longitude and Latitude in decimal degrees, and often an estimated accuracy in meters. Most apps just display these values as is, without considering formatting or number of significant digits. This blog post attempts to show how this could be done. I’ll use C# for this, but it should apply to any device, language and API out there.
Formatting
Some people think of longitude as the “X” in an ordinary flat X,Y the coordinate system and latitude as Y/Up/North. It’s technically not correct because this is not a flat coordinate system, but a ‘polar’ coordinate system - I do however understand this simplification and I think of it the same way internally when working with code that deals with any type of coordinate system. However, how things work internally and how they are displayed are two very different things. An example of this are date objects: They are usually stored and handled as a ‘tick’ number, but we never display it like that. Geographical coordinates are the same way. They have one way they are stored in memory, and a completely different way to be displayed.
First of all lets get the order out of the way: If you still think of longitude as the ‘x’ you probably want to display this value first. However it’s commonly agreed upon that latitude is displayed before longitude.
Next are negative coordinates. Instead of showing a latitude/longitude as for instance -117,34 you would write 117°W 34°N. So we prefix the coordinate with N/S and E/W depending on weather the coordinate is positive or negative. So in C# this could look like this:
char ns = lat < 0 ? 'S' : 'N'; //Southern or Northern hemisphere?
char ew = lon < 0 ? 'W' : 'E'; //Eastern or Western hemisphere?
string formatted = string.Format("{0}°{1} , {2}°{3}", Math.Abs(lat), ns, Math.Abs(lon), ew);
Now this is still decimal degrees. A more ‘proper’ format would be to use the degrees, minutes, seconds (DMS) format . Some people do prefer decimal degrees though, so you might want to make this a configurable option. But if you expect people to be using this coordinate to plot a position on a map, you are better off using DMS, since this is the format maps uses along its edge - and it also looks prettier. Degrees are denoted with a °, minutes with a single quote ' and seconds with a double quote ". For example 117°W 23' 12.34”
To make this conversion you will first show the integer part of the degrees. Take the remainder multiply by 60, and you’ll get the minutes. Lastly take the remainder of that and do the same, and you got the seconds (and you can display the seconds with decimals, but see the part on ‘accuracy’ next). Below is what that will look like in C#:
char ns = lat < 0 ? 'S' : 'N'; //Southern or Northern hemisphere?
char ew = lon < 0 ? 'W' : 'E'; //Eastern or Western hemisphere?
//Make positive
lon = Math.Abs(lon);
lat = Math.Abs(lat);
//Grab the part in front of the decimal
var majorLong = Math.Floor(lon);
var majorLat = Math.Floor(lat);
//the value after the decimal in minutes (*60)
var minorLong = (lon - majorLong) * 60;
var minorLat = (lat - majorLat) * 60;
//Minutes:
var minutesLong = Math.Floor(minorLong);
var minutesLat = Math.Floor(minorLat);
//Seconds:
var secondsLong = (minorLong - minutesLong) * 60;
var secondsLat = (minorLat - minutesLat) * 60;
string formatted = string.Format("{0}{1}°{2}'{3}\" {4}{5}°{6}'{7}\"",
ns, majorLat, minutesLat, secondsLat,
ew, majorLong, minutesLong, secondsLong);
Accuracy
Often I see a location displayed as for example -117.342817243 , 34.212381313. When I see this many digits I instantly think ‘oooooh that’s a very accurate location’. But this is very misleading. In college, several of our professors would fail our reports if the end result displayed more digits than the accuracy of the input data. The same thing applies here. If your GPS receivers accuracy is 1000m, how many digits should you display, and how many meters is one second?
First a little about the size and shape of earth: While earth is not a perfect sphere, it’s fairly close to an ellipsoid (this is still an approximation though). It’s widest at equator, and smallest (flattened) between the north and south pole. So in ellipsoid-speak the parameters are:
Semi-major axis: 6,378,137m
Semi-minor axis: 6,356,752.3142m
Mean radius (mR): 6,367,449m
So back to the question: How many meters is one second? This is pretty easy to determine for latitude, but unfortunately this is not a straightforward conversion for longitude, since this changes with the latitude. Let’s first start with the simpler latitude:
First we need the circumference of Earth along a meridian (a line that goes north/south) and Equator:
Circumference at Equator: 2 * Pi * 6,378,137 = 40,075,016 m
Circumference of a meridian : 2 * Pi * 6,356,752 = 39,940,652 m
For simplicity let's stick with a rough average of 40mio meters, since this is not going to really matter for the end result.
From that we get:
Horizontal length of one degree at Equator or along a meridian:
40,000,000 / 360 = 111,111m
Horizontal length of one second at Equator or along a meridian:
111111.111 / 60 minutes / 60 seconds = 31m
So from that we get that we should never display any decimals on seconds unless our accuracy is better than 31 meters. And we shouldn’t display more than one decimal unless the accuracy is 3m or better (which never happens with standard GPS equipment). Similarly if we are using decimal degrees instead of DMS for display, how much does the n'th digit matter at Equator or along a meridian?
5 digits: 0.000,01 * 40000000 = 400m
6 digits: 0.000,001 * 40000000 = 40m
7 digits: 0.000,000,1 * 40000000 = 4m
So in this case we will only show 7 digits if accuracy is better than 40m, and probably never more than 7 digits.
Latitude always goes along a meridian, so the number of significant digits doesn't ever change with your location. But the length of one degree at a longitude changes with the latitude you're at.
The radius of a longitude at a given latitude is: cos(latitude)*mR*2*PI.
At 34 north or south that would be: 33,168,021m. So here the number is roughly 3m instead of 4m, meaning you are more likely to show more digits on the longitude portion for the coordinate, the further north you go. In general practice however, this is not going to matter too much, since it only gets better. so to keep it simple we’ll just stick with the same conversion at all latitudes.
Bringing it all together
So let’s bring all this together into a C# ValueConverter you can use for binding against a GeoCoordinate object returned by the GeoCoordinateWatcher in .NET and Windows Phone. A converter parameter is used for choosing whether you want DMS or decimal degrees as output.
using System;
using System.Device.Location;
using System.Globalization;
using System.Windows.Data;
namespace CoordinateDisplay
{
/// <summary>
/// Converts a GeoCoordinate to Degrees-Minutes-Seconds
/// </summary>
public class CoordinateConverter : IValueConverter
{
private const double MeanEarthRadius = 6367449; //meters
private const double MeanEarthCircumference = 2 * Math.PI * MeanEarthRadius; //meters
public object Convert(object value, Type targetType, object parameter, CultureInfo culture)
{
if (value is GeoCoordinate)
{
var coord = value as GeoCoordinate;
if(coord.IsUnknown) return "Unknown";
double lat = coord.Latitude;
double lon = coord.Longitude;
if ((parameter is string) &&
string.Compare("decimal", parameter as string, StringComparison.OrdinalIgnoreCase) == 0)
//show as decimal degrees
{
var decimalsLat = 7;
var decimalsLon = 7;
int val = 4;
if (coord.HorizontalAccuracy > val * 100) decimalsLat = 5;
else if (coord.HorizontalAccuracy > val * 10) decimalsLat = 6;
val = (int)Math.Floor(Math.Cos(lat / 180 * Math.PI) * MeanEarthCircumference / 10000000d);
if (coord.HorizontalAccuracy > val * 100) decimalsLon = 5;
else if (coord.HorizontalAccuracy > val * 10) decimalsLon = 6;
return string.Format("{0}°,{1}°", Math.Round(lat, decimalsLat), Math.Round(lon, decimalsLon));
}
else //Show as degrees/minutes/seconds
{
char ns = lat < 0 ? 'S' : 'N'; //Southern or Northern hemisphere?
char ew = lon < 0 ? 'W' : 'E'; //Eastern or Western hemisphere?
//Make positive
lon = Math.Abs(lon);
lat = Math.Abs(lat);
//Grab the part in front of the decimal
var majorLong = Math.Floor(lon);
var majorLat = Math.Floor(lat);
//the value after the decimal in minutes (*60)
var minorLong = (lon - majorLong) * 60;
var minorLat = (lat - majorLat) * 60;
//Seconds:
var minutesLong = Math.Floor(minorLong);
var minutesLat = Math.Floor(minorLat);
//one digit accuracy on one second equals ~3m or better
//this changes with the latitude, but this is good enough for now
int decimals = 1;
if (coord.HorizontalAccuracy > 30)
decimals = 0; //With this accuracy we don't need to show sub-second accuracy
//Seconds:
var secondsLong = Math.Round((minorLong - minutesLong) * 60, decimals);
var secondsLat = Math.Round((minorLat - minutesLat) * 60, decimals);
return string.Format("{0}{1}°{2}'{3}\" {4}{5}°{6}'{7}\"",
ns, majorLat, minutesLat, secondsLat,
ew, majorLong, minutesLong, secondsLong);
}
}
return value;
}
public object ConvertBack(object value, Type targetType, object parameter, CultureInfo culture)
{
throw new NotSupportedException();
}
}
}
Here’s an example of using that in XAML where the datacontext is the GeoCoordinate:
<Grid>
<Grid.Resources>
<local:CoordinateConverter x:Name="dmsConverter" />
</Grid.Resources>
<StackPanel>
<TextBlock Text="Degrees minutes seconds:" />
<TextBlock Text="{Binding Converter={StaticResource dmsConverter}}" />
<TextBlock Text="Decimal degrees:" />
<TextBlock Text="{Binding Converter={StaticResource dmsConverter}, ConverterParameter=decimal}" />
<TextBlock Text="{Binding Path=HorizontalAccuracy, StringFormat=Accuracy: \{0:0\}m}" />
</StackPanel>
</Grid>
And what this can look like on a Windows Phone with different accuracies (notice the different number of digits):
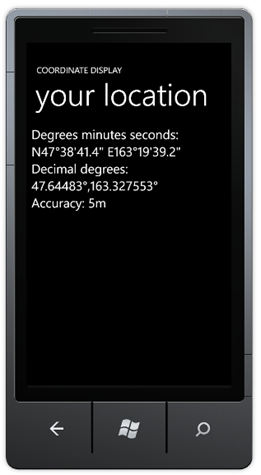
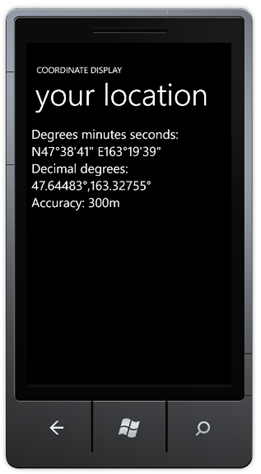
You can download this sample app here.